Creating our first Spring Web MVC Application
We are going take a look at how we can create a basic Spring Web MVC application. In order to develop a Spring Web MVC application, we need the following software and tools.
Java SE Development Kit 7 or later version Eclipse – Version: Neon Release (4.6) Maven 3.1.0 Apache Tomcat 8.0 (Servlet Container) Creating our first Spring MVC project
1.) In Eclipse. Go to File | New | Project; a New Project wizard window will appear. 2.) Select Maven Project under "Maven" and click on the Next button, as shown in the following screenshot:

3.) Now, a "New Maven Project" dialog window will be appeared; just leave the “Create a simple project (skip archetype selection)” checkbox (leave the default settings) and click on the Next button;

4.) Now, select an Archetype as “mavenarchetype-webapp” as shown in the below image and click ‘Next’.

5.) This wizard will ask you to specify “Group Id”, “Artifact Id” for your project; click next. Note: "Group Id" specifies the name of project group and the "Artifact Id" specifies the name of your maven based spring web mvc project that you want to create under the previously specified Group Id. Here, you could give any name to the Group Id and Artifact Id.

Now Eclipse is internally using Maven to create the project structure.
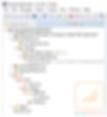
6.) Adding Spring MVC dependencies
We Spring libraries, as we are going to create Spring framework based web MVC application.
Open pom.xml in the eclipse editor window ( pom.xml is created under the root directory of the project itself) and then select " Dependencies " tab at the bottom of the eclipse editor window. Then, enter
Group Id as org.springframework Artifact Id as spring-webmvc Version as 4.2.5.RELEASE Select Scope as compile
Do the same for adding Servlet and JSTL Dependencies Group Id as javax.servlet Artifact Id as jstl Version as 1.2 select Scope as compile.
Group Id as javax.servlet Artifact Id as javax.servlet-api Version as 3.1.0 Scope as provided Save the pom.xml file.

After finishing step 6, you will see all the dependent jars of Spring web MVC, Servlet and JSTL are configured in your project, as shown in the following screenshot, under the Maven Dependencies library:
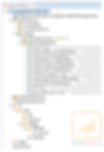
Adding Java compiler plugin and version properties in pom.xml
We need to add the Java compiler plugin to tell Maven which JDK version is used to compile your project. Again, open pom.xml file in the eclipse editor window. Add the following code in the POM.XML file, between the “project” element. <build> <plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.0</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
<finalName>SpringWebMVCApplication</finalName>
</build>
Save the “POM.XML” file and close it.
Writing source code for Spring Web MVC Application
As a first step, let's create a welcome /home page in our project to welcome our application users. So, whenever the user of your spring application enters the http:// localhost: 8080/ SpringWebMVCApplication/ URL on the browser, we would like to show a welcome page to the end user.
Adding a welcome page Create one welcome.jsp file, which is going to be a home page of our application: 1.) Create a WEB-INF/ jsp/ directory structure under the src/ main/ webapp/ directory; then create a welcome.jsp
<%@ page isELIgnored="false" %> <%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <html> <head> <meta http-equiv =" Content-Type" content =" text/ html; charset = ISO-8859-1" > <title> Welcome </title> </head> <body> <h1> ${greeting} </h1> <p> ${tagline} </p> </body >
</html >
2.) Create a class called HomeController under the javastrokes.com.webstore.controller package in the source directory src/ main/ java, and add the following code into it: Note: Create a folder called “java” under src/ main and then create a package hierarchy “javastrokes.com.webstore.controller” under src/ main/ java package com.packt.webstore.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; @Controller public class HomeController { @RequestMapping("/") public String welcome( Model model) { model.addAttribute("greeting", "Welcome to Web Store!"); model.addAttribute("tagline", "The one and only amazing webstore"); return "welcome"; } }
Configuring dispatcher servlet
Spring MVC framework provides pre defied servlet controller or front controller called dispatcher servlet (org.springframework.web.servlet.DispatcherServlet). The dispatcher servlet is what examines the incoming request URL and invokes the right corresponding controller method. In our case, the welcome method from the HomeController class needs to be invoked if we enter the http://localhost: 8080/SpringWebMVCApplication URL on the browser. So let's configure the dispatcher servlet for our project:
Create web.xml under the src/ main/ webapp/ WEB-INF/ directory in your project and enter the following content inside web.xml and save it:
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/webapp_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <servlet>
<servlet-name>spring</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> </servlet>
<servlet-mapping>
<servlet-name>spring</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping> </web-app>
Now create one more xml file called spring-servlet.xml under the same src/ main/ webapp/ WEBINF/ directory and enter the following content into it and save it:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <mvc:annotation-driven />
<context:component-scan base-package ="javastrokes.com.webstore.controller" />
<bean class ="org.springframework.web.servlet.view.InternalResourceViewResolver" > <property name ="prefix" value ="/WEB-INF/jsp/" />
<property name ="suffix" value =".jsp" />
</bean >
</beans >
Running the project Assume, you already configured the Tomcat web server in our eclipse, let's use Tomcat to deploy and run our project:
1.) Right-click on your project from Package Explorer and navigate to Run As | Run on Server.