Exception Handling in Java
- javastrokes
- Feb 20, 2017
- 3 min read
What is an Exception & The Causes of Exceptions ?
Exceptions are generated when a recognized condition, usually an error condition, occurs during the execution of a method. When an exception is generated, it is said to be thrown
An Exception is a run-time error
Causes normal program flow to be disrupted
An exception is thrown for the following reasons: • A throw statement was executed.
• An abnormal execution condition was synchronously detected by the Java Virtual Machine, namely: What Happens When an Exception Occurs?
● When an exception occurs within a method, the method creates an exception object and hands it off to the runtime system – Creating an exception object and handing it to the runtime system is called “throwing an exception” – Exception object contains information about the error, including its type and the state of the program when the error occurred ● When an appropriate handler is found, the runtime system passes the exception to the handler – An exception handler is considered appropriate if the type of the exception object thrown matches the type that can be handled by the handler – The exception handler chosen is said to catch the exception. ● If the runtime system exhaustively searches all the methods on the call stack without finding an appropriate exception handler, the runtime system (and, consequently, the program) terminates and uses the default exception handler
Exception Handling Process
When an exception occurs insdie a Java method, the method creates an Exception object and passes the Exception object to the JRE (in Java term, the method "throws" an exception). The Exception object contains the type of the exception, and the state of the program when the exception occurs. The JRE is responsible for finding an exception handler to process the Exception object. It searches backward through the call stack until it finds a matching exception handler for that particular class of Exception object (in Java term, it is called "catch" the exception). If the JRE cannot find a matching exception handler in all the methods in the call stack, it terminates the program.
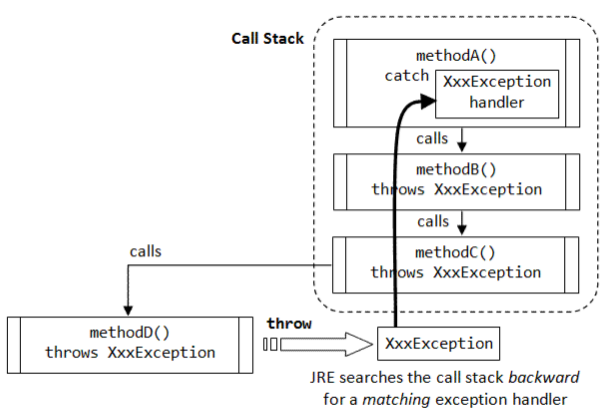
This process is illustrated as follows. Suppose that methodD() encounters an abnormal condition and throws a XxxException to the JRE. The JRE searches backward through the call stack for a matching exception handler. It finds methodA() having a XxxException handler and passes the exception object to the handler. Notice that methodC() and methodB() are required to "throws XxxException" upwards in order to compile the program.
Handling Exceptions Using try-catch Blocks
If a method is going to resolve an potential exception internally, the line of code that could generate the exception is placed inside a try block.
The try is used to define a block of code in which exceptions may occur
One or more catch blocks match a specific exception to a block of code that handles it.
There can be more than one catch block, each one marked for a specific exception class.
The exception class that is caught can be any class in the exception hierarchy, either a general (base) class, or a very specific (derived) class
If an exception occurs within a try block, execution jumps to the first catch block whose exception class matches the exception that occurred - and the remaining codes in the try block are skipped, if no exception occurs, then the catch blocks are skipped. Syntax: try { <code to be monitored for exceptions> } catch ( <ExceptionType1> <ObjName> ) { <handler if ExceptionType1 occurs> } ... …… } catch ( <ExceptionTypeN> <ObjName> ) { <handler if ExceptionTypeN occurs> }
Example
/* Using Multiple catch Blocks: -You can list a sequence of catch blocks, one for each possible exception. since the first one that matches is used and the others skipped. */ package javastrokes.com.trycatch; public class MultiCatchTest { public static void main(String[] args) { String num = "2"; int i1=5, i2 = 0; try { i1= Integer.parseInt( num ); System.out.println( i1 ); System.out.println( ); System.out.println( i1 / i2 ); System.out.println( "Statement inside the try block"); String names [] = {"A", "B", "C"}; System.out.println( names[6] ); System.out.println( "Last statement of the try block" ); } catch (ArithmeticException e ) { // 2nd catch block... //System.out.println(e); System.out.println("Divide by Exception occurred"); e.printStackTrace(); } catch (NumberFormatException e ) { System.out.println( e + " Give valid data"); e.printStackTrace(); } catch ( ArrayIndexOutOfBoundsException e ) { System.out.println(" Don't access the array values beyond its limit" ); System.out.println("final statement of your program after try catch"); System.out.println("final statement of your program after try catch"); } }
Comentarios