Java Inheritance Basics
- javastrokes
- Jan 4, 2017
- 2 min read
What is Inheritance ?
Inheritance allows you to use abstraction in a new way. Inheritance in Java lets you to create a new Abstract Data Type (ADT) by extending the existing Abstract Data Type. What is an Abstract Data Type (ADT) ?
For example, the Instrument class given below, represents an abstraction for a real-world Instrument. Here, the Instrument class is a ADT. When you create a class in Java, it lets you to create a new data type using an abstraction mechanism called data abstraction. The new data type is known as abstract data type (ADT). The data objects / instance variables / properties in ADT may consist of a combination of primitive data types and other ADTs. An ADT defines a set of operations / functions that can be applied to all its data objects.
The class "StringedInstrument" declared in the "StringedInstrument.java", shown below is a sub class, a child class, or a derived class of super type, a super class, a parent class, or a base class "Instrument" declared in the "Instrument.java".
Here, the existing abstraction (Instrument) is called a super type, a super class, a parent class, or a base class. The new abstraction (StringedInstrument) is called a sub type, a sub class, a child class, or a derived class. It is said that a sub type is derived (or inherited) from a super type; a super type is a generalization of a sub type; and a sub type is a specialization of a super type. The inheritance can be used to define new abstractions at more than one level. A sub type can be used as a super type to define another sub type and so on. Inheritance gives rise to a family of types arranged in a hierarchical form.
Instrument.java
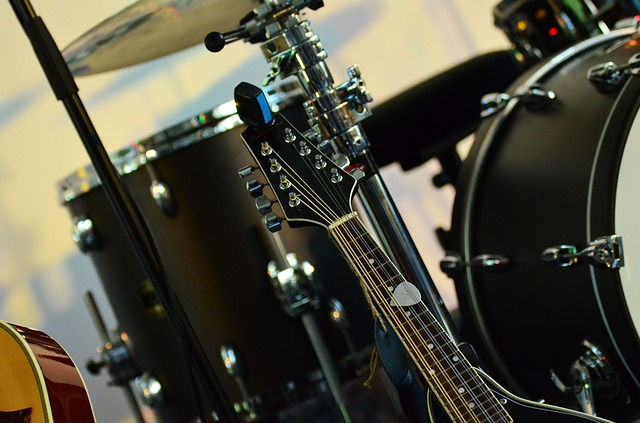
package abstractclass.music.instrument;
public abstract class Instrument { private String instrumentName; private String instrumentBrand; public Instrument (String instrumentName, String instrumentBrand) { this.instrumentName = instrumentName; this.instrumentBrand = instrumentBrand; } public String getInstrumentName() { return instrumentName; } public void setInstrumentName(String instrumentName) { this.instrumentName = instrumentName; } public String getInstrumentBrand() { return instrumentBrand; } public void setInstrumentBrand(String instrumentBrand) { this.instrumentBrand = instrumentBrand; } abstract public String instrumentBasicDetails(); // abstract method.... }
StringedInstrument.java
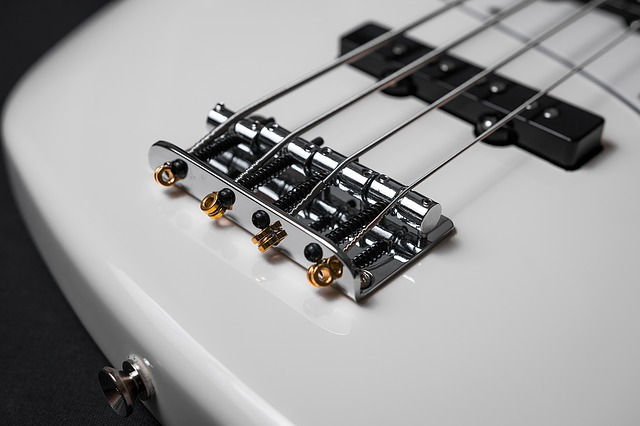
package abstractclass.music.instrument; public abstract class StringedInstrument extends Instrument { private int numberOfStrings; private String stringMade; public StringedInstrument (String instrumentName, String instrumentBrand, int numberOfStrings, String stringMade) { super (instrumentName, instrumentBrand); this.numberOfStrings= numberOfStrings; this.stringMade = stringMade; } public int getNumberOfStrings() { return numberOfStrings; } public void setNumberOfStrings(int numberOfStrings) { this.numberOfStrings = numberOfStrings; } public String getStringMade() { return stringMade; } public void setStringMade(String stringMade) { this.stringMade = stringMade; } @Override public String instrumentBasicDetails() { return "An Instrument Name: " + getInstrumentName() + " Brand: "+ getInstrumentBrand() + " " + "No of Strings: " + getNumberOfStrings()+ " stringMade: "+ getStringMade() +"\n"; } }
Comments